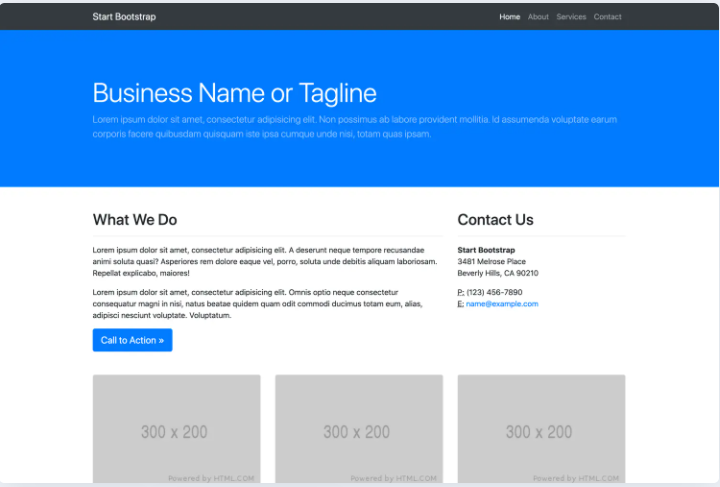
So, obviously a great place to start with my portfolio is how I built this website. As I showed this to people I had questions whether I used Wix or anything and while I have knowledge in WordPress, I chose to build this from scratch. However, I may decide on doing so since I’m finding part 2 (to be made) could be tricky to build it all from scratch and maybe a off the shelf set of code modules will do the trick both nicely and much more easily. Ultimately the purpose of the website is to show my skills and so don’t want to have someone or something do it, but, showing off skills in WordPress or Wix might not also be bad.
As I have built this up, I’ve tried to maintain my references and where I found different parts, to help me remember how I did it but also to give credit where credit is due! The first part was getting a UI, here I did choose to get something off the shelf bootstrap based as it’s already worked out all the particulars on multi-device support and offers a few simple designs. I decided upon this first starting point: “Business Frontpage”

Now that I had a template to work from, I next modified the CSS template to a colour scheme I liked. Ultimately I want something that is manageable and so I have chosen to dynamically load data where possibly requiring future changes via jQuery, PHP and loading from MySQL. Nicely, PHP was one of my first languages, I had started with javascript before that but found the whole thing as a starting language too difficult. This I believe is also way before the days of jQuery, I was 12 so 2004, PHP was much easier to learn at that time and I read a book incorporating both PHP and MySQL. Later I learned jQuery simply by doing and using tutorials/stackoverflow, so I don’t have references for how I did it since it was mostly off the top of my head and some corrections through google.
$(document).ready(function(){
$.ajax({
type: "GET",
url: 'content.php',
success: function(response)
{
var jsonData = JSON.parse(response);
for (let i = 0; i < jsonData.length; i++) {
//Since we only have one article - (to be updated, load all to cards).
$('#cards').append('<div class="col-md-4 mb-5"><div class="card h-100"><img class="card-img-top" src="' + jsonData[i].image + '" alt=""><div class="card-body"><h4 class="card-title">' + jsonData[i].title + '</h4><p class="card-text">' + jsonData[i].content.substring(0,80) + '</p></div><div class="card-footer"><a href="#" class="btn btn-primary">Find Out More!</a></div></div></div>');
}
}
});
});
This was the first dynamic section, which loaded the cards of my big 3 articles you see on the home page. So next let’s go to the php script, this is where I had a bit of trouble if I’m honest – getting a format where I provided an array of data back in JSON for my jQuery to interpret. We’ll discuss multi-dimensional later when I populate my navigation bar.
<?php
// Change this to your connection info.
$DATABASE_HOST = 'localhost';
$DATABASE_USER = '*********';
$DATABASE_PASS = '*********';
$DATABASE_NAME = 'MyContent';
// Try and connect using the info above.
$con = mysqli_connect($DATABASE_HOST, $DATABASE_USER, $DATABASE_PASS, $DATABASE_NAME);
if ( mysqli_connect_errno() ) {
// If there is an error with the connection, stop the script and display the error.
exit('Failed to connect to MySQL: ' . mysqli_connect_error());
}
// Attempt select query execution
$sql = "SELECT * FROM Project";
$myarray = array();
if($result = mysqli_query($con, $sql)){
if(mysqli_num_rows($result) > 0){
while($row = mysqli_fetch_array($result)){
array_push($myarray, array('title' => $row['Title'],'content' => $row['Content'],'image' => $row['MainImage'],'id' => $row['Index'],'thumb' => $row['Thumbnail'],'Lrgthumb' => $row['LrgThumbnail'],'TagIndexer' => $row['TagIndexer'],'PostedDate' => $row['PostedDate'],'LstEdit' => $row['LastEdited'],'likes' => $row['Likes'],'views' => $row['Views']));
}
echo json_encode($myarray);
} else {
echo "No records matching your query were found.";
}
} else {
echo "ERROR: Could not able to execute $sql. " . mysqli_error($con);
}
$con->close();
?>
So now we have both pieces let’s explain a few things. You’ll maybe notice my jQuery request is to “content.php”, sounds generic no? Well that’s the point, I want to re-use this for grabbing any data on articles and then I can filter it on the jQuery side. I also don’t want to pass it any request data to avoid SQL/JavaScript injection, I will keep my scripts to a one way calling to get data back.
So once we have our ajax call, we iterate over the returned JSON array and append to cards to create a new div of a card with the data embedded.
On the PHP side we simply connect to our database, get a SELECT of all our data and then iterate through this and do an array push with our array being the JSON that we later encode. In future, once I have more than 3 articles, I’ll move this script to call a “showcase.php” and have a unique table where I have a table of 3 which represents my top 3 articles I want to showcase. Like I said before, the PHP scripts will mostly do the work, here it’ll grab the secondary key’s on the showcase table and then it will grab the corresponding articles from that table.
And finally, as you can see from the array_push line, we have a table similar to this:

For my about page I wrote this up quite simply, later I plan on having this dynamically populate but only once I’ve decided on a good UI for editting the content from an admin side. The contact form I took from https://colorlib.com/wp/template/contact-form-v16/ and embedded this into the style of the page.
So now let’s lead up to the final parts, the actual articles themselves and how I populate the navigation to these.
$.ajax({
type: "GET",
url: 'categories.php',
success: function(response)
{
var jsonData = JSON.parse(response);
// loop the outer array
for (let i = 0; i < jsonData.length; i++) {
//Populate our menu
$('#projects').append('<a class="dropdown-item" href="category.php?name=' + jsonData[i].Name + '">' + jsonData[i].Name + '</a>');
}
}
});
This code snippet will sit on every page and simply loads what categories I have populated into my categories table into the dropdown menu of the navigation bar. I mentioned before I’m using bootstrap and the original template did not include a drop down menu, but it was simple to add as it is already part of bootstrap: Bootstrap Nav Bar.
Categories is a simple structure, three fields, ID, Name and Description, so I’ll spare the space of showing that. You can imagine the PHP is also very similar to the previous script. The only thing to note here is that in the append of the navbar, I have scripted in that the link includes POST data for the category.php page to know which category of articles to load when it loads.
The final segment therefore I’d like to cover to show some code is the categories pages themselves. For now, the actual articles themselves will be a similar flavour except that they shall use the POST data in the article.php page to extract the actual article in question and then show only this under contents under the container div. When I get round to building/integrating something like WordPress, this will need redoing so for now, I’m making it very simple so I can get straight to showcasing my work.
So the final part, when we load the categories page, we now need to load each article related to that category and display them one by one, after the other. Later I will build a max number of articles per page and a paging system, but I simply don’t require this right now as I have yet to write articles, don’t want to spend too much time on this before showcasing my skills!
We also want to load the header for the page, for that we have POST in the URL from before of our category name. For the name I simply embedded PHP to show this category name like so:
'<h1 class="display-4 text-white mt-5 mb-2">'<?php echo $_GET['name'];?>'</h1>
Similar to before, our categories.php script, like our content.php sends back all categories. So we can re-use this to auto populate our description. We can modify our above script by adding an additional statement into just this particular page, using a similar trick of embedding the POST data as directly above:
$.ajax({
type: "GET",
url: 'categories.php',
success: function(response)
{
var jsonData = JSON.parse(response);
// loop the outer array
for (let i = 0; i < jsonData.length; i++) {
//Populate our menu
$('#projects').append('<a class="dropdown-item" href="category.php?name=' + jsonData[i].Name + '">' + jsonData[i].Name + '</a>');
if('<?php echo $_GET['name'];?> = jsonData[i].Name){ //If our category matches, place the description in the header.
$('#desc').append(jsonData[i].Description);
}
}
}
});
Now for the articles themselves! Similar to directly above in fact, we’ll modify our previous ajax request to perform the same, populate the contents – this is only true for this page but also provides nice code re-usability and saved me alot of time.
$.ajax({
type: "GET",
url: 'content.php',
success: function(response)
{
var jsonData = JSON.parse(response);
//Iterate through our responses of articles available.
for (let i = 0; i < jsonData.length; i++) {
if(jsonData[i].category = '<?php echo $_GET['name'];?>){ //If our category matches, add the article.
$('#container').append(''<div class="row">'<div class="col-md-19 mb-5">'<h2>' + jsonData[i].title + ''</h2>'<hr>' + jsonData[i].content.substring(0,300) + ''<p>'</p>'<a class="btn btn-primary btn-lg" href="#">Learn More »'</a>'</div>'</div>');
}
}
}
});
Now almost to the finale was actually written up while writing up this article itself, you may have guessed it, the article page! Well, for now, here it is:
$.ajax({
type: "GET",
url: 'content.php',
success: function(response)
{
var jsonData = JSON.parse(response);
// user is logged in successfully in the back-end
// let's redirect
for (let i = 0; i < jsonData.length; i++) {
// user is logged in successfully in the back-end
// let's redirect
if(jsonData[i].id == "'<?php echo $_GET['id'];?>"){
$('#container').append(''<div class="row" style="overflow-wrap:break-word;width:100%;display:block;">'<div class="col-md-19 mb-5">' + jsonData[i].content + ''</div>'</div>');
$('#title').append(jsonData[i].title);
}
}
}
});
We once again, make it very simple, where we add our title into the main header and add our content to the container. Keeping it simples! This is of course, once we find a match to our article id which has been handed to use on page load by PHP. As you can imagine, once I had built up this basic workflow, it was more clean up (you may notice missing code fragments for instance here or other areas I just didn’t mention to!) and getting things more presentable. I won’t fixate on writing this any further until Part 2 as I think the basic workflow has been shown and an adequate developer can imagine how the rest took place.
Thanks for reading!