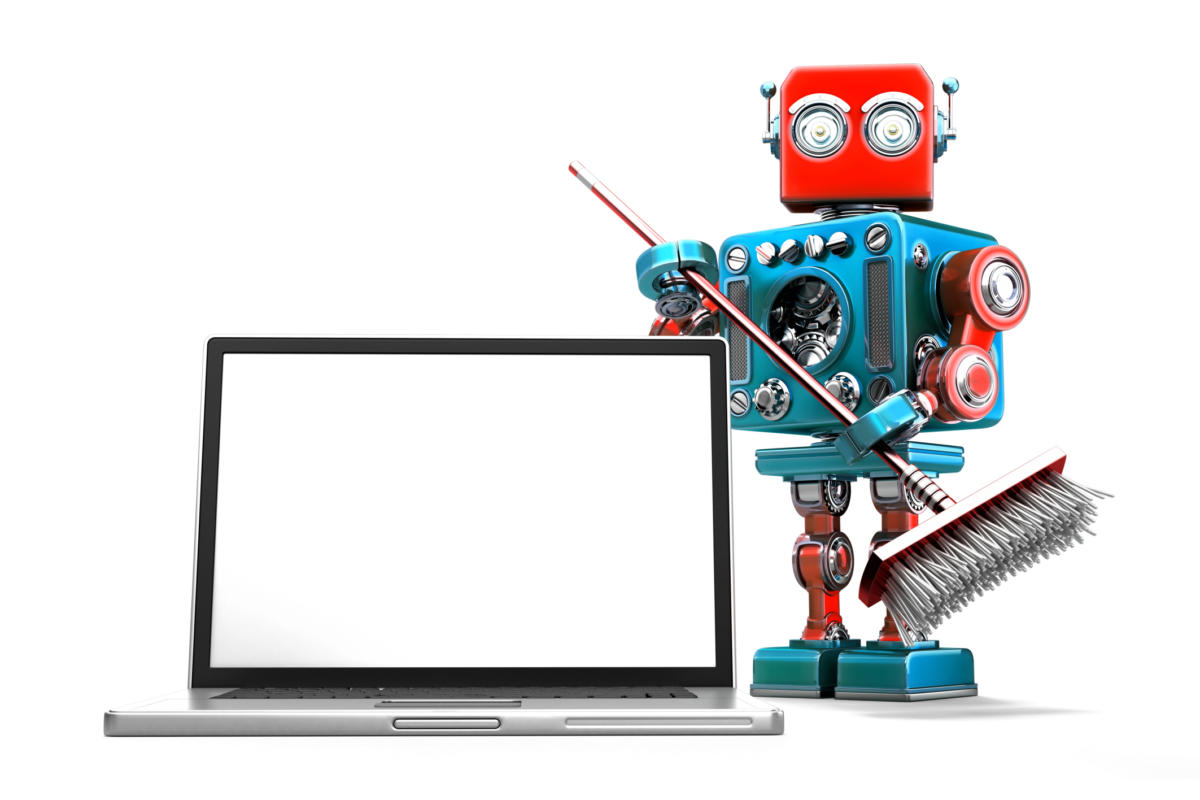
Thanks for sticking along my journey! In this article we’re going to cover some of the lower priority things I had, specifically the user experience (woops!). As is any engineers sin, I kept these till last as I saw them as odd jobs to get finished towards the end and when I was ready for beta/alpha testing with users.
So the first thing I noticed was that my code would overflow and in overflow it would extend the width, meaning pages on phones were very distorted.
I won’t reference a link as eventually, using multiple articles I settled on this approach:
pre {
white-space: pre-wrap;
word-wrap: keep-all; /* Internet Explorer 5.5+ */
}
.code {
overflow: auto;
width: 100%;
}
The footer fix was a bit more tricky, now my code generates from PHP I don’t think it’s absolutely necessary anymore but I’ll keep it anyway. I basically used this approach which worked well:
https://codepen.io/chriscoyier/pen/uwJjr
Image widths was fairly easy afterall, it was a simple matter of this CSS:
img {
display: block;
margin-left: auto;
margin-right: auto;
width:100%;
}
The contact us page is where it gets slightly interesting, it led no where! I double checked and the contact me form which I grabbed from https://colorlib.com/wp/template/contact-form-v16/ didn’t actually do anything. I know I didn’t mention this in my first article, apologies. One thing I found here was that it overrode some of my traditional bootstraps CSS so had odd looking fonts compared to other pages and was fairly noticeable. I used google chrome’s feature of inspect element and then removed links to CSS stylesheets, it was a mixed bag of results, some would destroy the whole style of the form if I removed them and others would get me closer to what I wanted. For those still persisting but couldn’t be removed, I then modified the CSS files directly to match the previous style before they get removed. Very tedious as you can imagine, looping through it all.
So back to the no actions problem now that that’s solved, how to solve this, well, the send button was my first issue. It was a button, not an input form, I tried modifying it to be an input form but then I lost my CSS and it looked awful. So instead I decided on an old school javascript solution. Here I changed the buttons destination from ‘#’ to:
<a class="btn btn-primary btn-lg" href="javascript:doSomething();">Send »</a>
Since it’s old style javascript as I call it (compared to jquery) it doesn’t need to be in a document.ready and I serialised the data in the form and sent this off to a PHP script to handle:
<script>
$(document).ready(function(){
});
function doSomething() {
window.location.replace("http://www.edward-jones.co.uk/email.php?"+ $('form').serialize());
}
</script>
So why this mixture? Well, javascript was good for hooking into a button and then serialising my form data. PHP was great for sending emails, simples really :). So as for the PHP side, this is where I had a bit of trouble but eventually found that my issue was more with godaddy than PHP, so here’s the code:
<?php
$message = $_GET['name'] . $_GET['email'] . $_GET['phone'] . $_GET['message'];
echo "Mail is sending...<p>";
mail("edjjones17@gmail.com","test",$message);
echo "Email has been sent!<p>Please wait 5 seconds to be redirected...";
header( "refresh:5;url=./index.html" );
?>
I did it like this because I found jQuery style to not give me any feedback. In future I’ll probably update this to not require a redirect off and on pages and do this in the background. This approach however let me echo the GET data and check what PHP was seeing from the serialised form. As you can see, it’s still a bit raw and sends the email with just the data. In future, I’ll fix this up, if you haven’t figured it out by now, when I say it, I do it!
So what was my issue with godaddy? Well, I hadn’t set up to allow my server and use my server as well, eventually I found this article which resolved things: https://uk.godaddy.com/community/Managing-Email/PHP-mail-not-sending/m-p/61549#M8505
We’re really steam rolling through this one aren’t we! Only two things left to do. An idea I had was to present my core values in my about page, this is because I do believe in a few principles from which you can draw a whole personality/attitude of success. I decided to present this by changing the header for starters and putting it here. I next thought, well, why not use bootstraps great ability to portion up segments into rows and columns. Then I figured, I’d have like any company does, an icon representation of the value, with a quote or description of the value just below. Well, I came up with this:
<!-- Header -->
<header class="bg-primary py-5 mb-5" style="width:100%">
<div class="container h-100">
<div class="row h-100 align-items-center justify-content-center">
<div class="col-sm justify-content-center">
<img src="win.jpg" width="100" class="center" />
</div>
<div class="col-sm justify-content-center">
<img src="download.png" width="100" class="center" />
</div>
<div class="col-sm justify-content-center">
<img src="teamwork.png" width="100" class="center" />
</div>
<div class="col-sm justify-content-center">
<img src="honest.png" width="100" class="center" />
</div>
</div>
<div class="row h-100 align-items-center justify-content-center">
<div class="col-sm justify-content-center corevalue">
<p></p><p> I never lose I either win or I learn
</div>
<div class="col-sm justify-content-center corevalue">
<p></p><p> Learning is earning
</div>
<div class="col-sm justify-content-center corevalue">
<p></p><p> Teamwork makes the dreamwork
</div>
<div class="col-sm justify-content-center corevalue">
<p></p><p> The badge of honesty is simplicity
</div>
</div>
</div>
</header>
You’ll notice the additional CSS as well, this was because it aligned left which didn’t look right, I also used a font generator to change my font style for the core values. Here is the CSS:
.corevalue{
font-family: "Trebuchet MS", Helvetica, sans-serif;
font-size: 14px;
letter-spacing: 2px;
word-spacing: 2px;
color: #FCFCFC;
font-weight: 700;
text-decoration: none;
font-style: normal;
font-variant: normal;
text-transform: none;
text-align:center;
}
.center {
display: block;
margin-left: auto;
margin-right: auto;
width: 50%;
}
And that was it really! I’m expecting as we progress articles on this whole subject of my web development that I can start more describing my challenges rather than “how it works”, if you’re a little confused, please refer to my previous parters to this.
And now to finish up with a bit of wordpress. I mentioned I didn’t like my index page dynamically loading data anymore since you could see the page load, then load again with the jQuery sections in cards. What I did to fix this was copy my code from category.php where I PHP load data and iterate through posts into the index.html, I then copied the jQuery alongside, replaced the PHP codes echo’ing, data grabbing and json request with the jQuery’s. When I was done, I deleted the jQuery snippets, renamed the index.html to index.php so it would understand the PHP code and voila!
<div class="row" id="cards">
<?php
$json = file_get_contents('http://www.edward-jones.co.uk/blog/wp-json/wp/v2/posts?per_page=3');
$posts = json_decode($json);
foreach ($posts as $p) {
echo '<div class="col-md-4 mb-5"><div class="card h-100"><img class="card-img-top" src="', $p->featured_image->size_medium, '" alt=""><div class="card-body"><h4 class="card-title">', $p->title->rendered;
echo '</h4><p class="card-text">', substr($p->excerpt->rendered,0,100);
echo '...</p></div><div class="card-footer"><a href="article.php?id=';
echo $p->id;
echo '" class="btn btn-primary">Find Out More!</a></div></div></div>';
}
?>
<!--<div class="col-md-4 mb-5">
<div class="card h-100">
<img class="card-img-top" src="https://placehold.it/300x200" alt="">
<div class="card-body">
<h4 class="card-title">Card title</h4>
<p class="card-text">Lorem ipsum dolor sit amet, consectetur adipisicing elit. Sapiente esse necessitatibus neque sequi doloribus.</p>
</div>
<div class="card-footer">
<a href="#" class="btn btn-primary">Find Out More!</a>
</div>
</div>
</div> -->
</div>
<!-- /.row -->
In my next article:
- Adding article meta data
- Modularising my code
- Clean up the contact email approach
- Protecting against security threats
- Search Engine Optimisation
- Feedback from users
- Hits per page
I am truly thankful to the holder of this web page who
has shared this impressive piece of writing