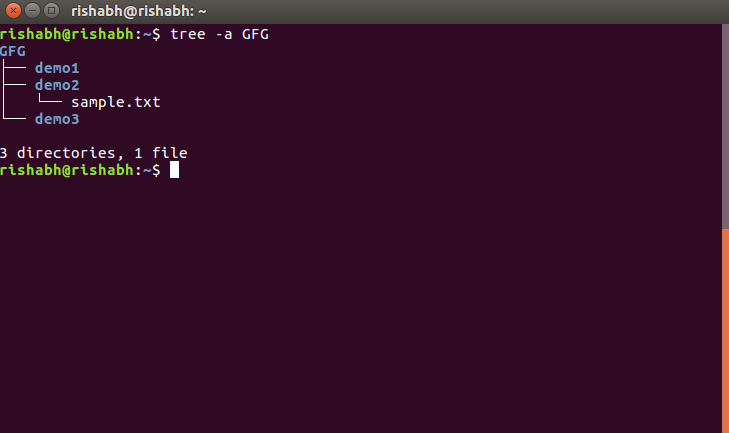
So I’m going to start with an easy and quick article today. Before I showed a pretty complex MARTe2.sh which relied on knowing exactly where each library of MARTe2 was and then adds these explicitly to the LD_LIBRARY_PATH so that the loader can find these.
One problem I have had with this is that I’m building MARTe2 into yocto so I first had to add a install script into the makefiles and for this, you could end up installing the libraries anywhere. So a more robust MARTe2.sh script was needed which worked for my yocto setup, whatever that may be and also for my other users in the team so we maintain a more universal MARTe2.sh script. Well for our team we have adapted the original. Let’s take a brief look at a part of the original shown in my first article.
#!/bin/bash
#Arguments -l LOADER -f FILENAME -m MESSAGE | -s STATE [-d cgdb|strace]
#-l LOADER=The Loader to use
#-f FILENAME=MARTe configuration file
#-m MESSAGE=Start message
#-s STATE=RealTimeApplication first state
#-d cgdb=Run with cgdb
#-d strace=Run with strace
#Run with cgdb or strace?
DEBUG=""
#Consume input arguments
while [[ $# -gt 1 ]]
do
key="$1"
case $key in
-l|--loader)
LOADER="$2"
shift # past argument
;;
-f|--file)
FILE="$2"
shift # past argument
;;
-m|--message)
MESSAGE="$2"
shift # past argument
;;
-s|--state)
STATE="$2"
shift # past argument
;;
-d|--debug)
DEBUG="$2"
shift # past argument
;;
--default)
DEFAULT=YES
;;
*)
# unknown option
;;
esac
shift # past argument or value
done
if [ -z ${MARTe2_DIR+x} ]; then echo "Please set the MARTe2_DIR environment variable"; exit; fi
if [ -z ${MARTe2_Components_DIR+x} ]; then echo "Please set the MARTe2_Components_DIR environment variable"; exit; fi
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:.
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:../../../../../Build/x86-linux/Examples/Core/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:Core/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_DIR/Build/x86-linux/Core/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/EPICSCA/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/LinuxTimer/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/LoggerDataSource/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/DAN/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/NI6259/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/NI6368/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/SDN/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/UDP/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/MDSWriter/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/RealTimeThreadSynchronisation/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/DataSources/FileDataSource/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/GAMs/IOGAM/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/GAMs/BaseLib2GAM/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/GAMs/ConversionGAM/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/GAMs/ConstantGAM/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/GAMs/FilterGAM/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/GAMs/StatisticsGAM/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/GAMs/WaveformGAM/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/Interfaces/BaseLib2Wrapper/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/Interfaces/SysLogger/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/Build/x86-linux/Components/Interfaces/EPICS/
echo $LD_LIBRARY_PATH
export LD_LIBRARY_PATH=$LD_LIBRARY_PATH
As I said, lots to unpack explicitly and assuming the directory structure. We can already see that a bunch of our lines are just in importing the library locations. So this is what we came up with in the team, the GAMs will be either .drv, .so, .a or .gam files and therefore we simply need to use the find function in linux to find each file and returns these. It has the same amount of lines roughly but it is dynamic to whereever they may be in the directory structure as long as we have the right MARTe2_DIR and MARTe2_Components_DIR:
#!/bin/bash
#Arguments -f FILENAME -m MESSAGE | -s STATE [-d cgdb|strace]
MDS=0
DEBUG=""
INPUT_ARGS=$*
RESIDUALS=""
while test $# -gt 0
do
case "$1" in
-f|--file)
CONFIG="$2"
FQCONFIG=$(realpath "$(pwd)/$CONFIG")
echo "config $1 $2"
echo "fqconfig $1 $FQCONFIG"
shift 2
echo "args remaining $#"
;;
-d|--debug)
DEBUG="$2"
echo "DEBUG $1 $2"
shift 2
echo "args remaining $#"
;;
-mds)
MDS=1
echo "MDS: $1"
shift
echo "args remaining $#"
;;
*)
echo "Default: $1"
RESIDUALS="$RESIDUALS $1"
echo "RESIDUALS : $RESIDUALS"
shift
echo "args remaining $#"
;;
esac
#shift
done
echo "Parsed the input args, residuals are"
echo "$INPUT_ARGS"
echo "and dollar star is now $RESIDUALS"
if [ -z ${MARTe2_DIR+x} ]; then
export MARTe2_DIR=~/Projects/MARTe2-dev/MARTe2
fi
if [ -z ${MARTe2_Components_DIR+x} ]; then
export MARTe2_Components_DIR=~/Projects/MARTe2-components
fi
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_DIR/Core/
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$MARTe2_Components_DIR/
#######################################
# Expand LD_LIBRARY_PATH for MARTe2_DIR
#######################################
m2_libs=$(find -L $MARTe2_DIR \
-name '*.so' -printf ' %h\n' \
-o -name '*.a' -printf ' %h\n' \
-o -name '*.gam' -printf ' %h\n' \
-o -name '*.drv' -printf ' %h\n' \
| sort -u | \
while read dir
do
printf ":%s" "$dir"
done)
#echo "m2_libs is"
#echo "$m2_libs"
#exit 54
export LD_LIBRARY_PATH=${LD_LIBRARY_PATH}${m2_libs}
##################################################
# Expand LD_LIBRARY_PATH for MARTe2_Components_DIR
##################################################
m2_component_libs=$(find -L $MARTe2_Components_DIR \
-name '*.so' -printf ' %h\n' \
-name '*.a' -printf ' %h\n' \
-name '*.gam' -printf ' %h\n' \
-name '*.drv' -printf ' %h\n' \
| sort -u | \
while read dir
do
printf ":%s" "$dir"
done)
export LD_LIBRARY_PATH=${LD_LIBRARY_PATH}${m2_component_libs}
Admittedly this isn’t the system I setup, I simply reused but think this is worthy of sharing, it’s been adapted a few times. I would show our overall script but it probably shows things about our system which I shouldn’t, or isn’t releasable yet anyway. But nevertheless you can now adapt your script with this system.
Short but sweet article!