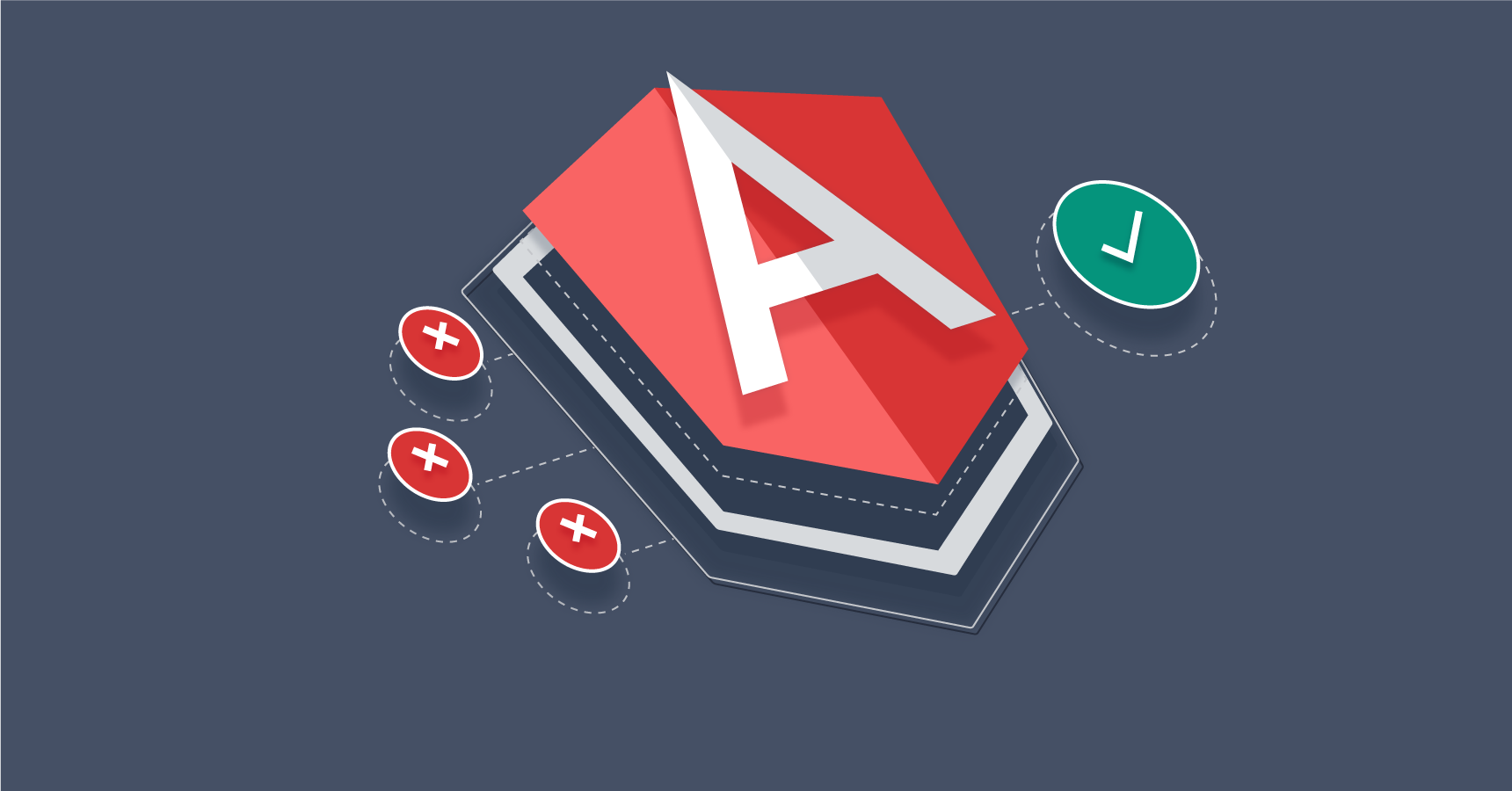
Okay so only two things left since part 3. This being, getting the subscribe component working and removing any console.log’s we implemented while debugging. The final part being a simple grep and possibly sed even with a regex. The first being a bit more complex, we need a component that appears on click and disappears when done.
Let’s start by creating our component:
ng generate component subscribe
Since this can be added anywhere we should add it to our application html:
<div id="container">
<app-subscribe *ngIf="isOn = true"></app-subscribe>
<app-navbar></app-navbar>
<router-outlet></router-outlet>
<app-footer></app-footer>
</div>
I use isOn as a variable inside my subscribe component based on this:
https://stackoverflow.com/a/62465641/14860365
I then simply copy and paste my subscribes template HTML into the component HTML and finally modify my subscribe button on the navbar component like so in the HTML:
<a class="nav-link" (click)="subscribeClick()">Subscribe</a>
I implement the event in the navbar but not in subscribe just yet to reclose everything, I then rebuild and take a look and unfortunately:

Well that’s a bit odd, oh yep, forgot to implement our CSS didn’t we. So once I copy that across and rebuild… I get nothing now.
I also noticed a new behaviour, if you navigate to a different tab, the subscribe div remains. So we’ll need to have navbar also trigger a close event when that happens. Anyway, once I’ve applied the CSS it looks as though my subscribe div now is completely gone even though we select it. This is however actually because we used javascript to create a sort of animation where it goes from zero height to some height:
function myFunction(){
// Animate the div element. A duration is set to 500 milliseconds.
$("#subscribe").animate({ width: '40%', height: '350px' }, 100);
$('#subscribe').show(); // Also show the div element.
}
What we’re learning here is how events work in angular, you may note before I could have implemented a pipe in angular for my highlight code functionality but didn’t as the article I used suggested, I’m simply unfamiliar with pipes and it seemed like it would be easier to not learn this right now and just copy and paste the code into where it was specifically needed.
But now let’s learn how to animate. Here I pull up two resources:
https://angular.io/guide/animations
https://angular.io/api/animations/animate
Okay so let’s start by updating our app typescript files as mentioned and then move onto the Subscribe component.
Now I started running into the issue that the subscribe component doesn’t have access to the trigger variable we use on the top-level. How to solve this? Well, unfortunately, let’s abandon the event approach, I found something similar:
https://stackoverflow.com/a/52414942/14860365
Given my navbar only has one real responsibility here (or two – when we route to close the subscribe panel) then this may be a better and easier approach while also consolidating all the functionality to the subscribe component with little mess or fuss.
So this is what my new app.component.html looks like:
<div id="container">
<app-subscribe #subscribe></app-subscribe>
<app-navbar [subscribe]="subscribe"></app-navbar>
<router-outlet></router-outlet>
<app-footer></app-footer>
</div>
Now before I go on to the navbar, I want to create some functions for my subscribe component to accommodate this methodology:
import { Component, OnInit } from '@angular/core';
import {
trigger,
state,
style,
animate,
transition,
} from '@angular/animations';
@Component({
selector: 'app-subscribe',
animations: [
trigger('openClose', [
state('open', style({
height: '350px',
width: '40%',
opacity: 1,
display: 'block',
})),
state('closed', style({
height: '0',
width: '0',
opacity: 0,
display: 'none',
})),
transition('open => closed', [
animate('0.1s')
]),
transition('closed => open', [
animate('0.1s')
]),
]),
],
templateUrl: './subscribe.component.html',
styleUrls: ['./subscribe.component.css']
})
export class SubscribeComponent implements OnInit {
constructor() { }
ngOnInit(): void {
}
isOpen = false;
toggle() {
this.isOpen = !this.isOpen;
}
open() {
this.isOpen = true;
}
close() {
this.isOpen = false;
}
}
And my subscribe.component.html:
<div class="subscribe" [@openClose]="isOpen ? 'open' : 'closed'" id="subscribe">
<span id='close'>x</span>
<div class="design">
<form class="formy" id="sub">
<div class="container">
<h2>Subscribe to my newsletter</h2>
<p>Subscribe to get a weekly email walking through the different articles I've posted in the week.</p>
</div>
<div class="container" style="background-color:white">
<input type="text" class="texti" placeholder="Name" name="name" id="name" required>
<input type="text" class="texti" placeholder="Email address" name="mail" id="email" required>
<label style="display:none;">
<input type="checkbox" class="check" checked="checked" name="subscribe" style="display:none;"> Weekly Newsletter
</label>
</div>
<div class="container">
<input type="submit" class="submit" value="Subscribe">
</div>
</form>
</div>
</div>
And in my navbar.component.ts:
import { Component, OnInit } from '@angular/core';
import { WordPressService, Category } from '../wordpress.service';
import { Input } from '@angular/core';
import { SubscribeComponent } from "../subscribe/subscribe.component";
@Component({
selector: 'app-navbar',
templateUrl: './navbar.component.html',
styleUrls: ['./navbar.component.css'],
})
export class NavbarComponent implements OnInit {
@Input() subscribe: SubscribeComponent;
rows: any;
constructor(public wpService: WordPressService) { }
ngOnInit(): void {
console.log("Here");
const req = this.wpService.getCategories();
req.subscribe(
(result: Array<Category>) => {
console.log('success', result);
this.rows = result
},
(error: any) => {
console.log('error', error);
});
}
subscribeClick() {
this.subscribe.open();
}
}
Okay so now I do get some functionality, the subscribe does appear when we press subscribe like so:
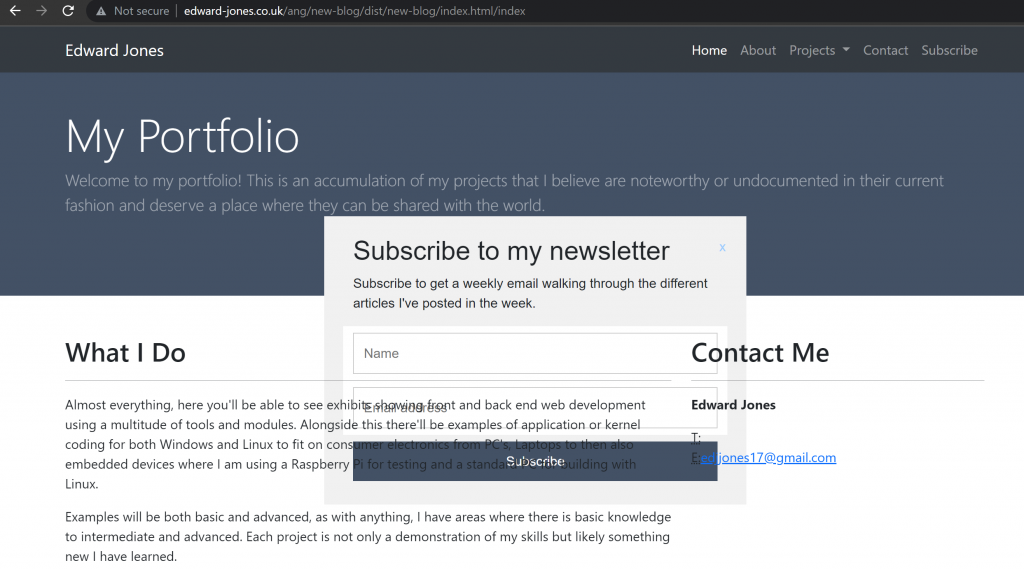
As you can see though, it seems to have half it’s opacity. It also seems like it’s z-index isn’t right and is behind the overall content while still being displayed. Playing around with the CSS in the google chrome inspect feature I found that this was primarily the z-index causing the issue – not opacity. So now I add this to the components css file and hey presto, it works now :).
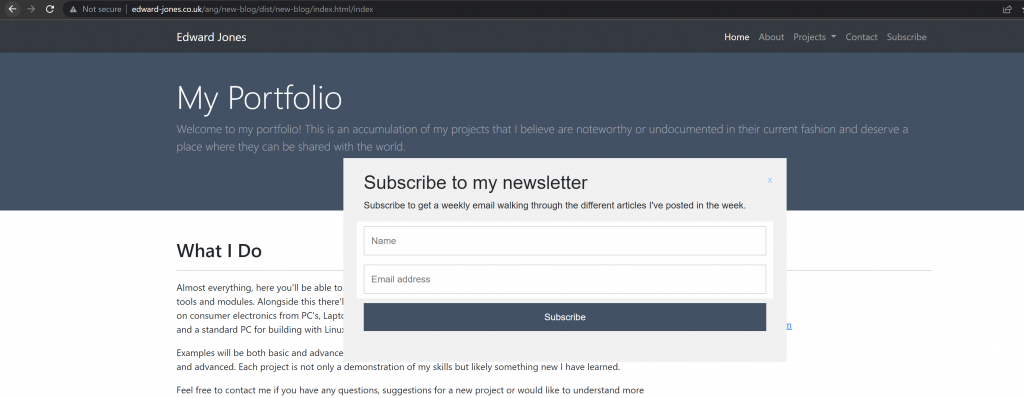
Now we just need to apply what we know from before:
- The close button should trigger the close function
- The subscribe button should grab the form data and send this to our readerService to send a post request
- the navbar should when routing, also trigger a function within navbar to close the subscribe if open.
All of these things we know, from before except one. So I won’t go into all this as it would just be repeating myself.
So for my final part – the third item in our list, I realised that any route link should just close subscribe and by chance happened upon this method:
https://stackoverflow.com/a/42453471/14860365
I basically implemented this method and in the “your code goes here” do, this.close();. Of which, this works perfectly.
Okay let’s wrap up some things, why did I do all this? Especially given I had a perfectly working website as it was:
- To learn angular for my next project
- To consolidate my website into a more naturally manageable method. As you may note, I had a article on modularising my website, this is just implementing that in a proper framework.
- To provide a way in order to later setup a gitlab instance with a CI/CD mechanism for my web development.
So that’s it, now I just need to preserve any PHP files I use in my application – let’s transition these to assets. Then I need to simply rebuild and finally copy my dist folder to my root public_html folder. And we’re live!
It’s funny, in my initial starting of my project I was simply copying and pasting and when I looked at full fledged examples I got daunted at the complexity of even the root directory code. But now, my own website seems to be on the same level of number of component imports and complexity – so, this exercise has been useful, I no longer find Angular to be a daunting task to undertake.