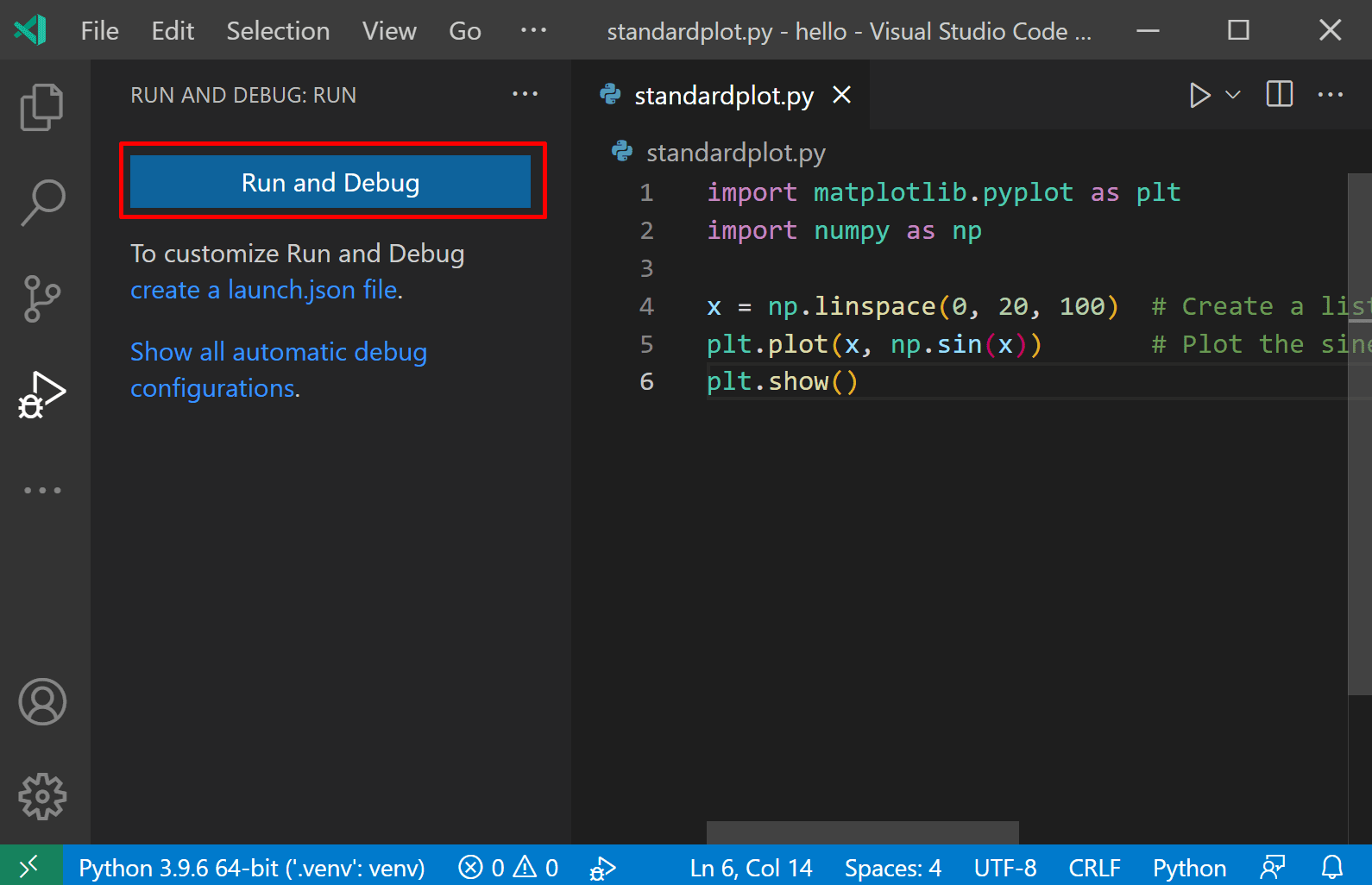
A recent project of mine involved a 3-4 layer hierarchy of multiple repo’s using submodules in Gitlab. I’ve recently seen some articles pushing the idea of singular repo’s for such projects for the simplicity of debugging and thought, hmmm… I’ve got a pretty good way around this.
So, what makes it tricky to debug is really that python has site-packages and your submodules are typically installed in there prior to debugging some new issue you’ve stumbled upon.
Then when your debugger starts up with your application it’s already using the site-packages rather than the actual local files in the submodules. This means you can’t debug within those local files, you might be able to somehow get your debugger to open the site package equivalents but when you modify code there it just gets all confusing… and more difficult to track source code control wise so, just don’t.
The issue is that your application at first entry point starts with the imports. As soon as it says “import this dependency” python goes through it’s PYTHON_PATH (just like $PATH) variable and iterates each directory in there to look for the import you have specified.
The first of which being, the site-packages directory. You might see where I’m going with this. Let’s say you have a submodule structure like so:
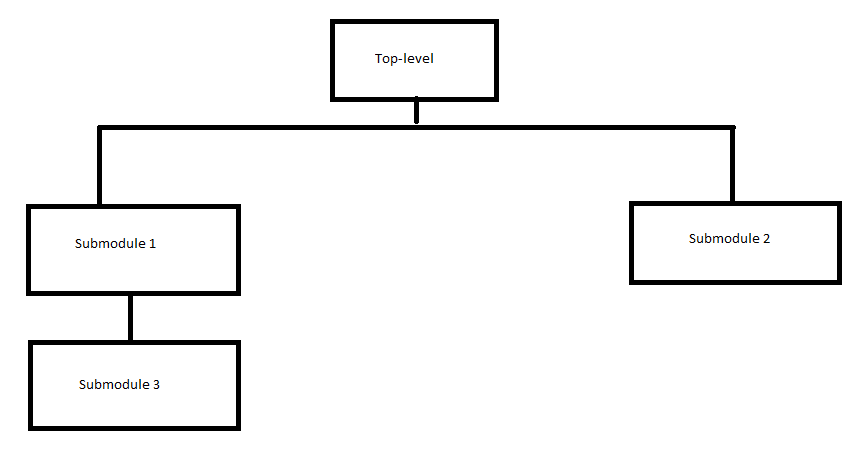
So take your application entry point is __main__.py or whichever file you’re running when you click run in visual code. Then insert at the start of this file while you want to be debugging:
import sys
sys.path.insert(0, r"./submodule 1/)
sys.path.insert(0, r"./submodule 2/)
sys.path.insert(0, r"./submodule 1/submodule 2)
And it’s that easy, now you can take this anyway, you could put this in a file called debug_mode.py and instead import that:
import .debug_mode
Or encapsulate the whole thing and use a variable to define whether you are debugging (maybe even put it into your .bashrc):
import os
import sys
# Check if DEBUG_MODE environment variable is set and equals "YES"
if os.environ.get('DEBUG_MODE') == 'YES':
# Your debug code here
print("Debug mode is enabled.")
sys.path.insert(0, r"./submodule 1/)
sys.path.insert(0, r"./submodule 2/)
sys.path.insert(0, r"./submodule 1/submodule 2)
And voila, now for your .bashrc in your terminal do:
echo "export DEBUG_MODE=YES" >> ~/.bashrc
source ~/.bashrc
From herein, whenever you run, it’ll be in debug mode for just you or any other developer you have update and source their .bashrc.
It is possible to also add this variable in Windows, very simply via:
https://superuser.com/questions/949560/how-do-i-set-system-environment-variables-in-windows-10